Creating health system
Hi, the next update will be bigger, and its release will be a little delayed.
But today, instead of update, I would like to show a couple of ideas on how to create a health system in the game.
Please note: i am not a native english speaker, so yeah, this text may contain some errors.
I come with 3 ideas:
- Simple health system(Only heal/get damage)
- Health system with part damage modificator(Headshot,leg shot, etc)
- Coming from the second, every part have own hp.
We all started with something and usually people add a health system directly to the character.
Something like this,
and so for each character of a monster, etc. We are lazy people and every time we dont want to write the same thing.
Therefore, we will create a special class that will do everything for us if we need to interact with the health of the object. Okay let's start.
SIMPLE HEALTH SYSTEM
First of all, create new script and name it like "HealthManager" or something like that.
Next, delete all code.
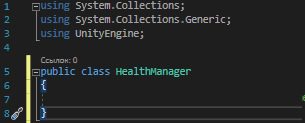
Also you can delete "using UnityEngine".
Now let's write standart code what we usually using,
public class HealthManager { private float health = 100f; //Class contructor, we need it for create custom health like monster with 200hp and etc public HealthManager(float h) { health = h; } //void for character damage public void HealthDamage(float damage) { health -= damage; } //void for character heal public void HealthHeal(float heal) { health += heal; } //for display out current health public float OurHealth() { return health; } //if we don't want call OurHealth() every time, we can just check is player dead public bool IsDead() { return health <= 0; } }
Okay, all done. What's next ? Now let's put this heath manager to our character.
And try it out.
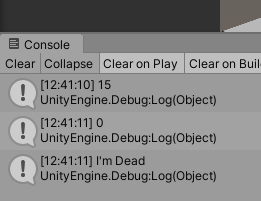
Great! All things worked. Now you can just attach HealthManager class to all your monsters and make them suffer.
Health system with part damage modificator(Headshot,leg shot, etc)
Now let's modify our system to be more realistic. For this we need separate all body parts, and think about how many damage will we recieve.
public class HealthManager { //Create bodyparts public Health hp; public BodyPart head; public BodyPart torso; public BodyPart rightHand; public BodyPart leftHand; public BodyPart rightLeg; public BodyPart leftLeg; //Set damage modificator for out parts and how many hp we have. public HealthManager(float health, float headMod, float torsoMod, float rightHandMod, float leftHandMod, float rightLegMod, float leftLegMod) { hp = new Health(health); head = new BodyPart(headMod, hp);//We need link our health with part, so we provide Health object for it torso = new BodyPart(torsoMod, hp); rightHand = new BodyPart(rightHandMod, hp); leftHand = new BodyPart(leftHandMod, hp); rightLeg = new BodyPart(rightLegMod, hp); leftLeg = new BodyPart(leftLegMod, hp); } } public class BodyPart { float damageModificator;//our damageModificator Health hp; public BodyPart(float damageMod,Health health)//Constuctor, we don't use it themself { damageModificator = damageMod; hp = health; } public void DoDamage(float damage) { hp.health -= damage * damageModificator; } } public class Health//Health class, need for link all parts with one hp bar { public float health; public Health(float hp) { health = hp; } public float ShowHp() { return health; } }
OMG,looks scary... You may ask, how i can remember what i need write in code ? For this Visual Studio(and i think others) have hints
Okay,let's try it out.
Before start,do some calculations. We make headshots do 90% damage,so 30*0.9=27. Now check it on practice
100-27=73.Yes that's correct.
Every part have own hp
Just change Health class to float,yes that's all
public class HealthManager { //Create bodyparts float hp; public BodyPart head; public BodyPart torso; public BodyPart rightHand; public BodyPart leftHand; public BodyPart rightLeg; public BodyPart leftLeg; //Set damage modificator for out parts and how many hp we have. public HealthManager(float health, float headMod, float torsoMod, float rightHandMod, float leftHandMod, float rightLegMod, float leftLegMod) { hp = health; head = new BodyPart(headMod, hp);//We need link our health with part, so we provide Health object for it torso = new BodyPart(torsoMod, hp); rightHand = new BodyPart(rightHandMod, hp); leftHand = new BodyPart(leftHandMod, hp); rightLeg = new BodyPart(rightLegMod, hp); leftLeg = new BodyPart(leftLegMod, hp); } } public class BodyPart { float damageModificator;//our damageModificator public float hp; public BodyPart(float damageMod,Health health)//Constuctor, we don't use it themself { damageModificator = damageMod; hp = health; } public void DoDamage(float damage) { hp -= damage * damageModificator; } }
Get Endalaus
Endalaus
Roguelite game in building
Status | In development |
Author | AdmiralSlowpoke |
Genre | Survival |
Tags | 3D, Crafting, Dungeon Crawler, First-Person, Singleplayer, Thriller, Voxel |
Languages | English, Russian |
More posts
- New update 0.0.2.3Mar 24, 2020
- New update 0.0.2.2Mar 23, 2020
- New update 0.0.2.1Mar 23, 2020
- New update 0.0.2.0Mar 22, 2020
- New update 0.0.1.7-0.0.1.8Mar 18, 2020
- New update 0.0.1.6Mar 14, 2020
- New update 0.0.1.5Mar 13, 2020
- Creating glock modelMar 11, 2020
Leave a comment
Log in with itch.io to leave a comment.